文章摘要:在C#中实现软件加密可以通过多种方式来完成。以下是一种常见的方法,使用加密算法(如AES或RSA)来加密你的软件代码或关键数据。
C#中使用AES算法实现软件加密
发布时间:2023-09-10 作者:小沈子 分类: C#
在C#中实现软件加密可以通过多种方式来完成。以下是一种常见的方法,使用加密算法(如AES或RSA)来加密你的软件代码或关键数据。
以下是一个简单的示例,使用AES算法来加密和解密字符串:
using System;
using System.IO;
using System.Security.Cryptography;
using System.Text;
public class AesExample{
public static void Main()
{
try
{
// 原始数据
string original = "这是需要加密的原始数据";
Console.WriteLine("Original: " + original);
// 创建一个随机的密钥和初始化向量
using (Aes myAes = Aes.Create())
{
byte[] encrypted = EncryptStringToBytes_Aes(original, myAes.Key, myAes.IV);
string roundtrip = DecryptStringFromBytes_Aes(encrypted, myAes.Key, myAes.IV);
Console.WriteLine("Encrypted: " + BitConverter.ToString(encrypted));
Console.WriteLine("Decrypted: " + roundtrip);
}
}
catch (Exception e)
{
Console.WriteLine("Error: {0}", e.Message);
}
}
static byte[] EncryptStringToBytes_Aes(string plainText, byte[] Key, byte[] IV)
{
byte[] encrypted;
using (Aes aesAlg = Aes.Create())
{
aesAlg.Key = Key;
aesAlg.IV = IV;
ICryptoTransform encryptor = aesAlg.CreateEncryptor(aesAlg.Key, aesAlg.IV);
using (MemoryStream msEncrypt = new MemoryStream())
{
using (CryptoStream csEncrypt = new CryptoStream(msEncrypt, encryptor, CryptoStreamMode.Write))
{
using (StreamWriter swEncrypt = new StreamWriter(csEncrypt))
{
swEncrypt.Write(plainText);
}
encrypted = msEncrypt.ToArray();
}
}
}
return encrypted;
}
static string DecryptStringFromBytes_Aes(byte[] cipherText, byte[] Key, byte[] IV)
{
string plaintext = null;
using (Aes aesAlg = Aes.Create())
{
aesAlg.Key = Key;
aesAlg.IV = IV;
ICryptoTransform decryptor = aesAlg.CreateDecryptor(aesAlg.Key, aesAlg.IV);
using (MemoryStream msDecrypt = new MemoryStream(cipherText))
{
using (CryptoStream csDecrypt = new CryptoStream(msDecrypt, decryptor, CryptoStreamMode.Read))
{
using (StreamReader srDecrypt = new StreamReader(csDecrypt))
{
plaintext = srDecrypt.ReadToEnd();
}
}
}
}
return plaintext;
}
}
这个例子只是为了说明加密和解密的过程。在实际应用中,你需要保管好你的密钥和初始化向量,以便在解密时使用。另外,这个例子中的密钥和初始化向量是随机生成的,实际应用中你可能需要一个安全的密钥和IV生成方式。同时,AES算法也有多种模式和填充方式,你可能需要根据实际需求来选择合适的模式和填充方式。此外,如果你需要对文件进行加密,那么你需要处理文件的读写和内存管理,这比处理字符串要复杂一些。
点击排行
标签云
-
C#
seo
SQLserver
IIS
.NET
PC支付
编程语言
SSL
程序员
微信小程序
VS
网站关键词排名
jQuery
服务器
个人博客
网站建设
301
https
免费模板
响应式
自动备份
数据库
Queue队列
.net8
http
KOL
C#集合
.NET框架
命名空间
面向对象编程
异常处理
异步编程
设计模式
编程学习网站
百度分享js
关键词研究工具
网页加载速度
外部链接优化
个人网站
WPF
数据库优化
winform
UI
编程
Ngrok
内网穿透
开源框架
NanUI
网站
清明节
C#接口
装潢设计
网页
挖呀挖
幸福
鸡汤
小沈子
超实用工具箱
Layui
51劳动节
C#面试题
疫情
.NETCore
微信接口
数组去重
404页面
保存图片
QQ
标签打印
icon图标
博客模板
html
生成img
nginx
签到
2023跨年
快捷方式
Web前端框架
JavaScript
TortoiseSVN
VS2019
数据库自动同步工具
Serv-U
营销网站
赞助打赏
- 支付宝扫码
- 微信扫码
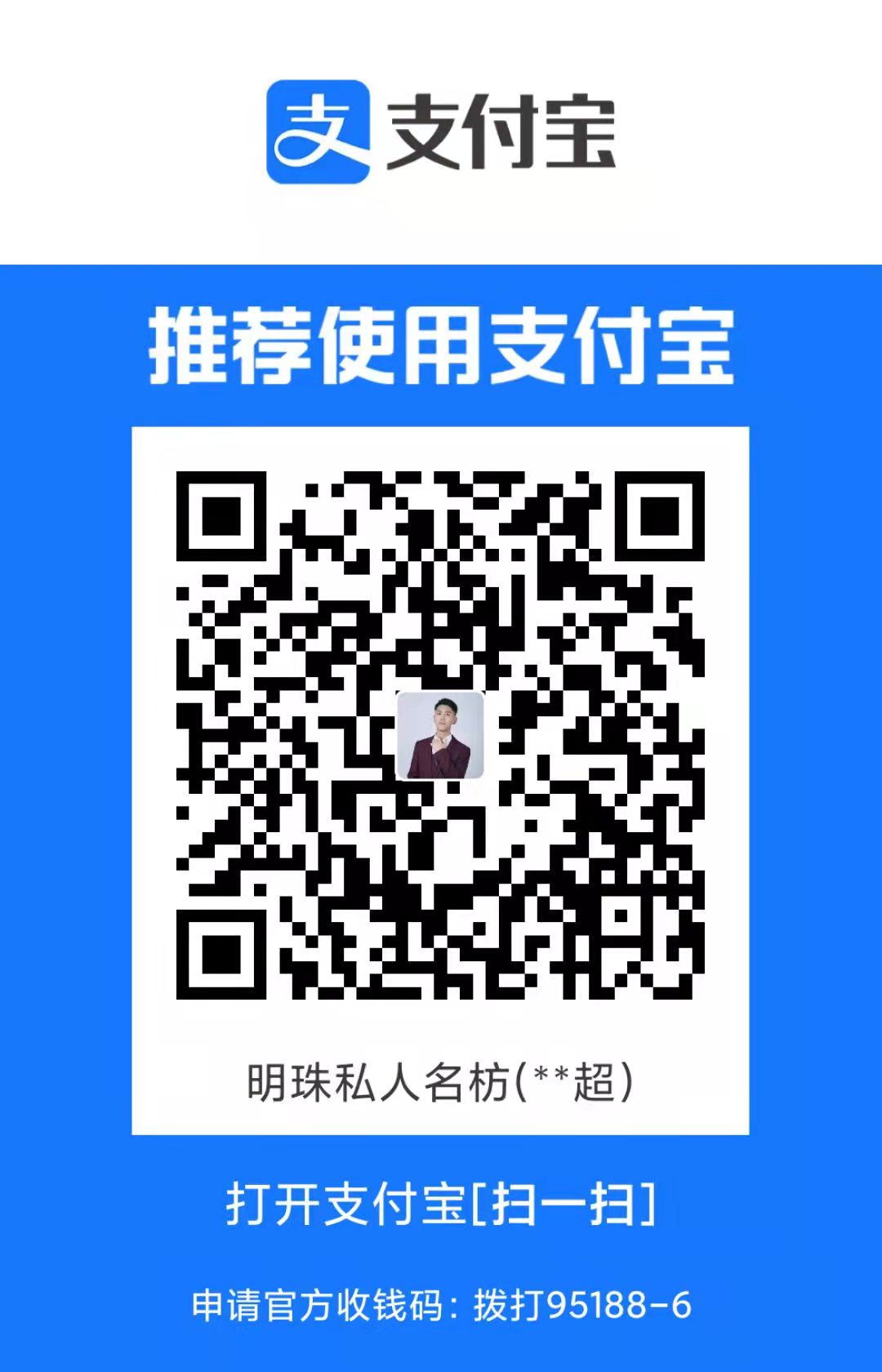
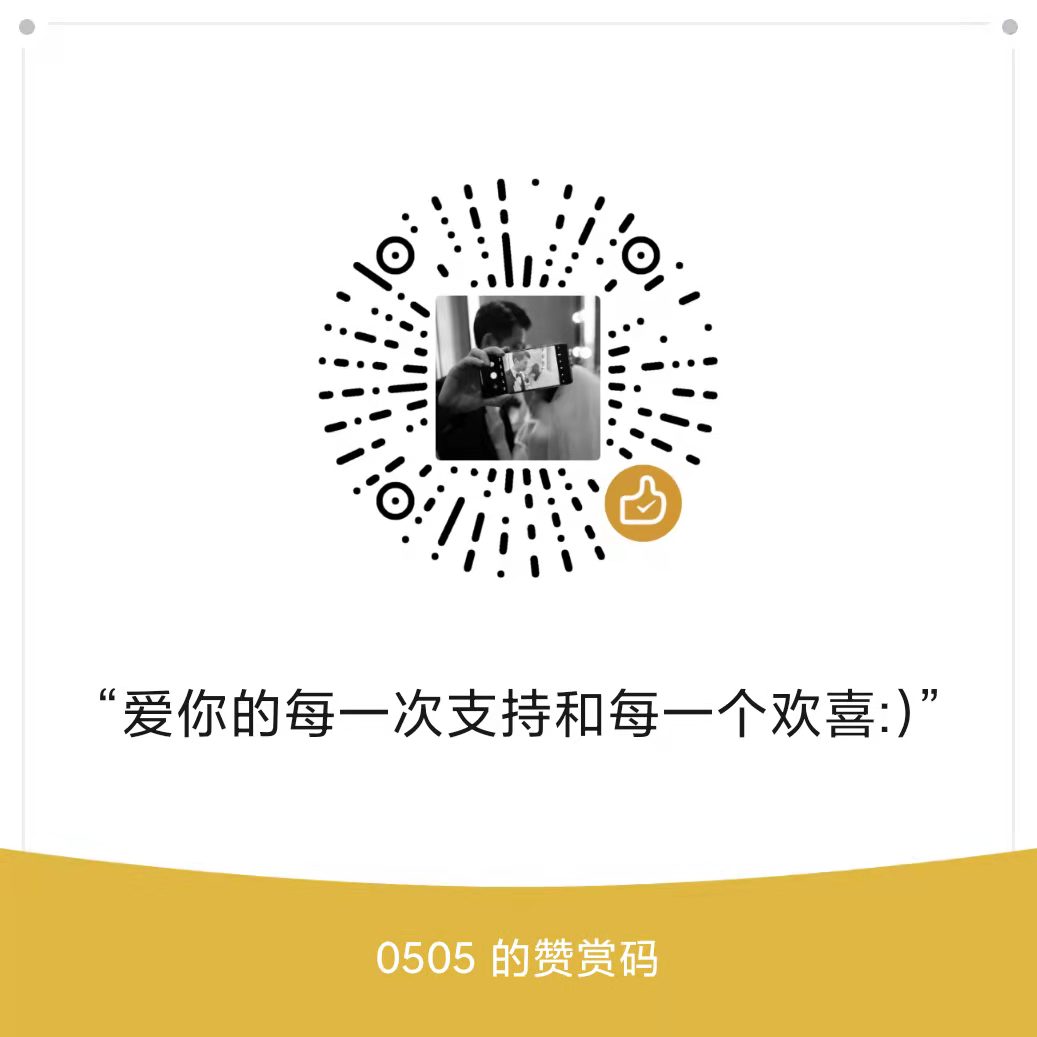